🔥 个人主页: 黑洞晓威
😀你不必等到非常厉害,才敢开始,你需要开始,才会变的非常厉害
543. 二叉树的直径
给你一棵二叉树的根节点,返回该树的 直径 。
二叉树的 直径 是指树中任意两个节点之间最长路径的 长度 。这条路径可能经过也可能不经过根节点 root
。
两节点之间路径的 长度 由它们之间边数表示。
解题思路
- 递归计算深度: 使用递归计算每个节点的左子树深度和右子树深度,然后返回以该节点为根节点的子树的深度。
- 更新直径: 在递归过程中更新直径,直径值为左子树深度加右子树深度的最大值。
- 结果返回: 最终得到的直径就是二叉树的直径。

代码实现
class Solution {int diameter = 0;public int diameterOfBinaryTree(TreeNode root) {calculateDepth(root);return diameter;}private int calculateDepth(TreeNode node) {if (node == null) {return 0;}int leftDepth = calculateDepth(node.left);int rightDepth = calculateDepth(node.right);diameter = Math.max(diameter, leftDepth + rightDepth);return Math.max(leftDepth, rightDepth) + 1;}
}
101. 对称二叉树
给你一个二叉树的根节点 root
, 检查它是否轴对称。
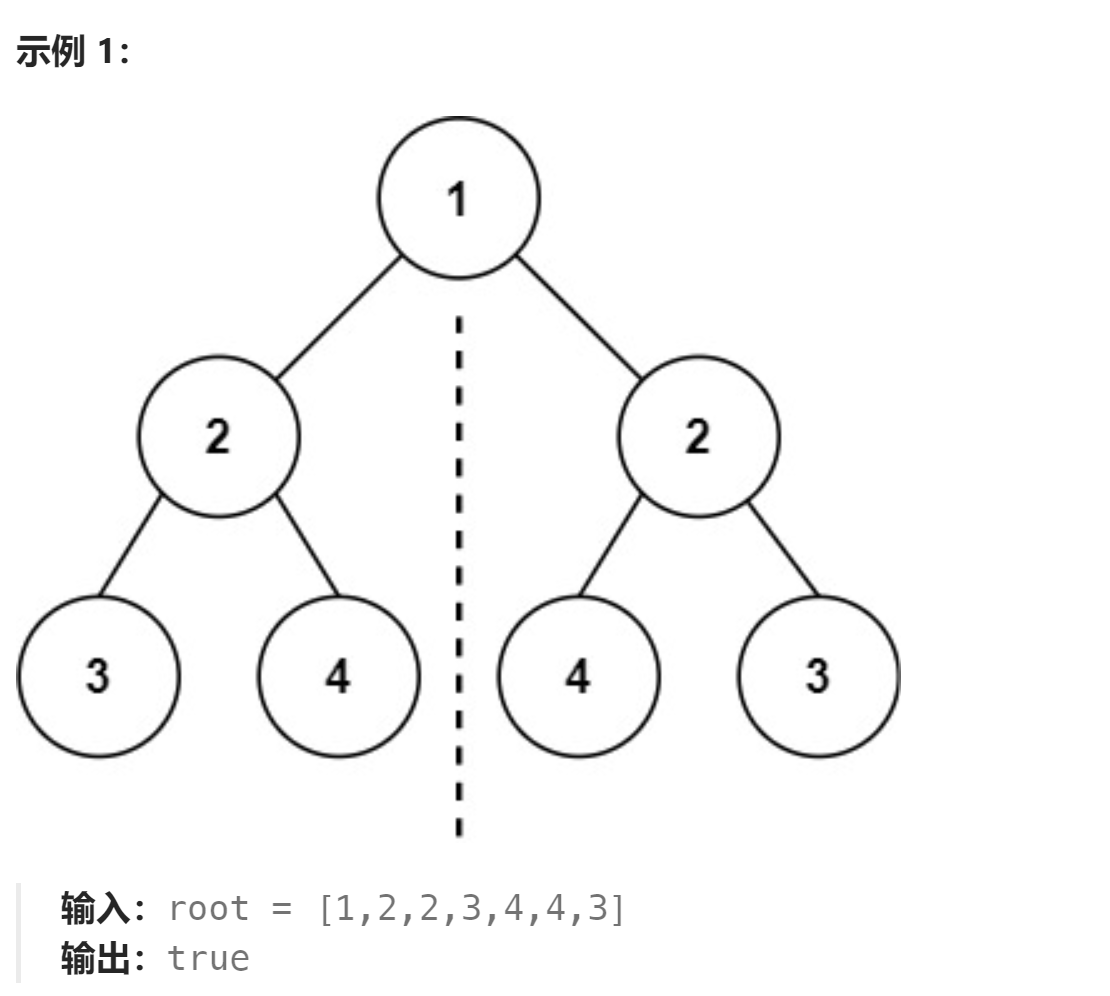
解题思路
- 定义一个递归函数,比较左右子树是否镜像对称。
- 递归地判断左右节点是否镜像对称,即左子树的左节点与右子树的右节点比较,左子树的右节点与右子树的左节点比较。
- 如果当前节点为空,返回 true。如果左右子树其中一个为空或者值不相等,返回 false。
代码实现
class Solution {public boolean isSymmetric(TreeNode root) {if (root == null) {return true;}return isMirror(root.left, root.right);}private boolean isMirror(TreeNode left, TreeNode right) {if (left == null && right == null) {return true;}if (left == null || right == null) {return false;}return (left.val == right.val)&& isMirror(left.left, right.right)&& isMirror(left.right, right.left);}
}